- August 6, 2024
- Posted by: Editor
- Categories: Gemini, Google
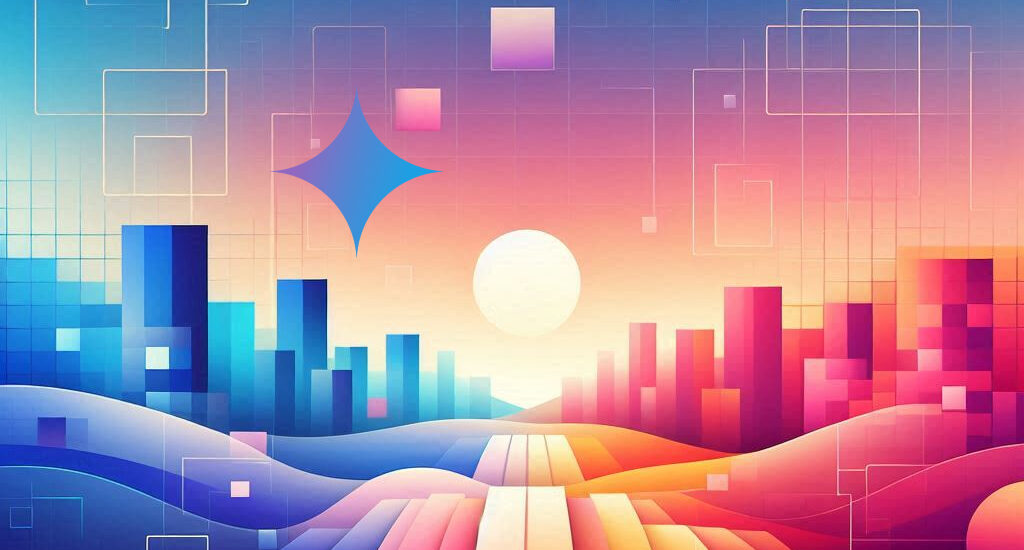
While there’s no official Google Gemini API library for PHP it’s very easy to connect to the API using basic PHP code and curl. Let’s build a simple function that will use curl to accept a prompt and display a response from Gemini.
Prerequisites
- IDE or your favorite Text Editor e.g. Visual Studio Code or Notepad++
- Gemini API Key
- PHP 7.4+
To create an API key you will need to go to https://aistudio.google.com/app/u/1/apikey and click on Create API key
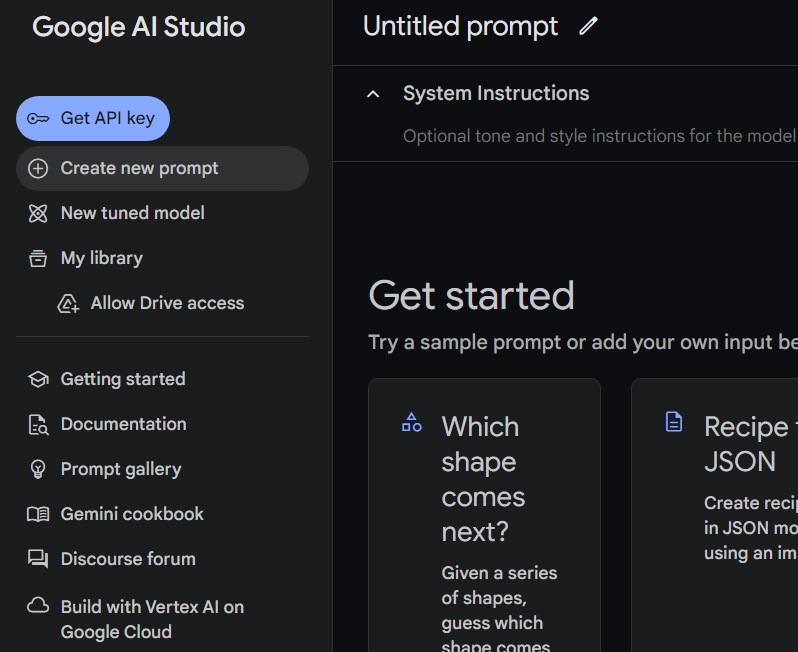
If you have a pre-existing project in your Google Cloud projects you can use the search bar to select it.
Alternatively, you can create an API key by clicking the blue Create API key in new project.
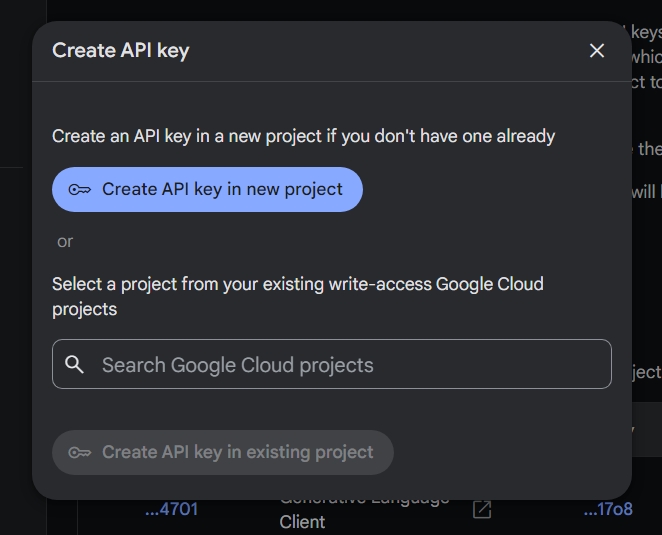
Copy the API key that is generated and displayed on screen.
Now create your project folder. We can call this folder gemini for testing.
Create your index.php file and add the following code. Paste your API key into the GEMINI_API_KEY definition. Note that Gemini offers different models depending on your needs or preferences. You can take a look at those over here. For the example below we will be using Gemini 1.5 Flash Latest.
<?php
define('GEMINI_API_KEY','enter your api key here');
define('MODEL','gemini-1.5-flash-latest');
define('BASEURL', 'https://generativelanguage.googleapis.com/v1beta');
function generateContent($textPrompt)
{
$text = filter_var($textPrompt, FILTER_SANITIZE_STRING);
//combine the base url with preferred model and api key
$url = sprintf("%s/models/%s:generateContent?key=%s",BASEURL,MODEL,GEMINI_API_KEY);
$data = [
"contents" => [
[
"role" => "user",
"parts" => [
[
"text" => $text
]
]
]
]
];
$jsonData = json_encode($data);
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, ['Content-Type: application/json']);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $jsonData);
$response = curl_exec($ch);
if (curl_errno($ch)) {
echo 'Request error: ' . curl_error($ch);
} else {
print_r(json_decode($response));
}
curl_close($ch);
}
generateContent('What is PHP');
Now what you’ll notice is that we get a ton of extra information back in the response. So we’ll have to parse the returned object and extract the pieces of information that we want. To do we can add the following code.
//print_r(json_decode($response));
replace the previous code here with the following
if (isset($response->candidates[0]->content->parts[0]->text)) {
$text = $response->candidates[0]->content->parts[0]->text;
echo $text;
} else {
echo "No response returned";
}
You’ll notice that the response is delivered in markdown. To further enhance the response we can use a markdown library to convert the response into html or tell Gemini directly to return the data already formatted in html by slightly adjusting our prompt.
Here’s a sample response using the alternative prompt.
generateContent('What is PHP? Format the response in simple html.');
Produces…
<!DOCTYPE html>
<html>
<head>
<title>What is PHP?</title>
</head>
<body>
<h1>What is PHP?</h1>
<p>PHP stands for **Hypertext Preprocessor**. It is a widely-used, open-source scripting language designed for web development.</p>
<p>Here are some key features of PHP:</p>
<ul>
<li><strong>Server-side scripting:</strong> PHP code runs on the server, generating HTML that is then sent to the user's browser.</li>
<li><strong>Dynamic content:</strong> PHP allows you to create dynamic web pages that change based on user input, database information, or other factors.</li>
<li><strong>Database connectivity:</strong> PHP integrates seamlessly with popular databases like MySQL, PostgreSQL, and Oracle.</li>
<li><strong>Open source and free:</strong> PHP is free to use and distribute, making it an attractive choice for both individuals and businesses.</li>
<li><strong>Large community and support:</strong> PHP has a huge community of developers, providing ample resources, documentation, and support.</li>
</ul>
<p>Overall, PHP is a powerful and versatile language that is well-suited for a wide range of web development tasks.</p>
</body>
</html>
Gemini is an exciting alternative to OpenAI products and offers a generous free tier which makes it a compelling offering for quick hobby projects or for testing various ideas. You can further improve this code by adding vision recognition which allows us to upload images for analysis or adding features that use ajax to build a real-time chatbot. In our follow up article we’ll delve into how to add vision capabilities as an enhancement.